来自:chuckfang.com/2019/11/13/Async
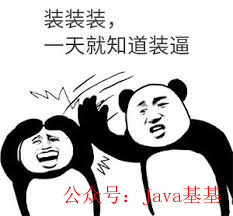
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableAsync;
@SpringBootApplication
@EnableAsync
public class AsyncdemoApplication {
public static void main(String[] args) {
SpringApplication.run(AsyncdemoApplication.class, args);
}
}
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Component;
import java.util.Random;
/**
* @author https://www.chuckfang.top
* @date Created on 2019/11/12 11:34
*/
@Component
public class Task {
public static Random random = new Random();
@Async
public void doTaskOne() throws Exception {
System.out.println("开始做任务一");
long start = System.currentTimeMillis();
Thread.sleep(random.nextInt(10000));
long end = System.currentTimeMillis();
System.out.println("完成任务一,耗时:" + (end - start) + "毫秒");
}
@Async
public void doTaskTwo() throws Exception {
System.out.println("开始做任务二");
long start = System.currentTimeMillis();
Thread.sleep(random.nextInt(10000));
long end = System.currentTimeMillis();
System.out.println("完成任务二,耗时:" + (end - start) + "毫秒");
}
@Async
public void doTaskThree() throws Exception {
System.out.println("开始做任务三");
long start = System.currentTimeMillis();
Thread.sleep(random.nextInt(10000));
long end = System.currentTimeMillis();
System.out.println("完成任务三,耗时:" + (end - start) + "毫秒");
}
}
@SpringBootTest
class AsyncdemoApplicationTests {
public static Random random = new Random();
@Autowired
Task task;
@Test
void contextLoads() throws Exception {
task.doTaskOne();
task.doTaskTwo();
task.doTaskThree();
Thread.sleep(10000);
}
}
❝ 开始做任务三 开始做任务二 开始做任务一 完成任务一,耗时:4922毫秒 完成任务三,耗时:6778毫秒 完成任务二,耗时:6960毫秒 ❞
@SpringBootTest
class AsyncdemoApplicationTests {
public static Random random = new Random();
@Test
void contextLoads() throws Exception {
doTaskOne();
doTaskTwo();
doTaskThree();
Thread.sleep(10000);
}
@Async
public void doTaskOne() throws Exception {
System.out.println("开始做任务一");
long start = System.currentTimeMillis();
Thread.sleep(random.nextInt(10000));
long end = System.currentTimeMillis();
System.out.println("完成任务一,耗时:" + (end - start) + "毫秒");
}
@Async
public void doTaskTwo() throws Exception {
System.out.println("开始做任务二");
long start = System.currentTimeMillis();
Thread.sleep(random.nextInt(10000));
long end = System.currentTimeMillis();
System.out.println("完成任务二,耗时:" + (end - start) + "毫秒");
}
@Async
public void doTaskThree() throws Exception {
System.out.println("开始做任务三");
long start = System.currentTimeMillis();
Thread.sleep(random.nextInt(10000));
long end = System.currentTimeMillis();
System.out.println("完成任务三,耗时:" + (end - start) + "毫秒");
}
}
❝ 开始做任务一 完成任务一,耗时:9284毫秒 开始做任务二 完成任务二,耗时:8783毫秒 开始做任务三 完成任务三,耗时:943毫秒 ❞
https://www.baeldung.com/spring-async
是这样说的:❝ First – let’s go over the rules – @Async has two limitations:
it must be applied to public methods only self-invocation – calling the async method from within the same class – won’t work The reasons are simple – 「the method needs to be *public*」 so that it can be proxied. And 「self-invocation doesn’t work」 because it bypasses the proxy and calls the underlying method directly. ❞